You find forms everywhere in a data-driven application. Forms, as a rule, need some validation on the client-side to nudge users to fill in data in line with what we want. These validation are easy to implement in Vue.
Consider an example that includes Vuetify for styling the UI -
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
<template>
<div>
<v-form ref="form" v-model="valid">
<v-layout row wrap>
<v-flex xs12></v-flex>
<v-flex xs12>
<v-text-field
v-model="userId"
label="Full Name"
:rules="[rules.required, rules.min8Chars]"
></v-text-field>
</v-flex>
<v-flex xs12>
<v-text-field
v-model="userEmail"
label="Enter your e-mail"
:rules="[rules.required, rules.email]"
></v-text-field>
</v-flex>
<!-- not used. demonstrates that user cannot submit form unless valid
<v-flex xs12 class="pt-3 text-xs-right">
<v-btn flat outline @click="validateAndDoStuff">Validate</v-btn>
</v-flex> -->
</v-layout>
</v-form>
</div>
</template>
|
Above is a simple form with two fields - user-id and email. We have specified the validation rules applicable for the fields, and we define those rules in data
.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
<script>
export default {
data() {
return {
valid: true,
rules: {
min8Chars: (value) => value.length >= 8 || "Min. 8 characters",
required: (value) => !!value || "Required.",
email: (value) => {
const pattern = /^(([^<>()[\]\\.,;:\s@"]+(\.[^<>()[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
return pattern.test(value) || "Invalid e-mail.";
},
},
};
}, // data()
methods: {
validateAndDoStuff() {
if (this.$refs.form.validate()) {
// do stuff
alert("Valid!");
}
},
},
};
</script>
|
If you now try to enter invalid values, the form refuses to submit and shows error messages.
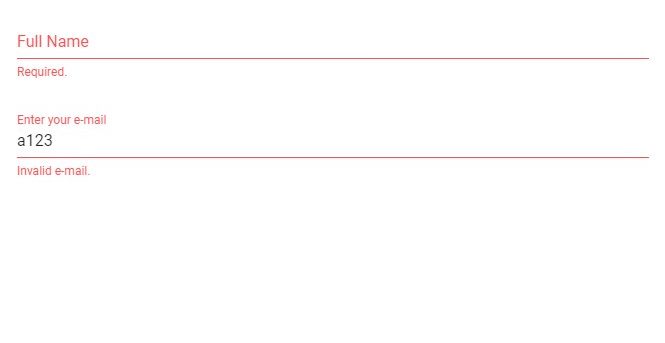
Explore popular ways to do form validation in Vue -